Getting Started With Automated Testing
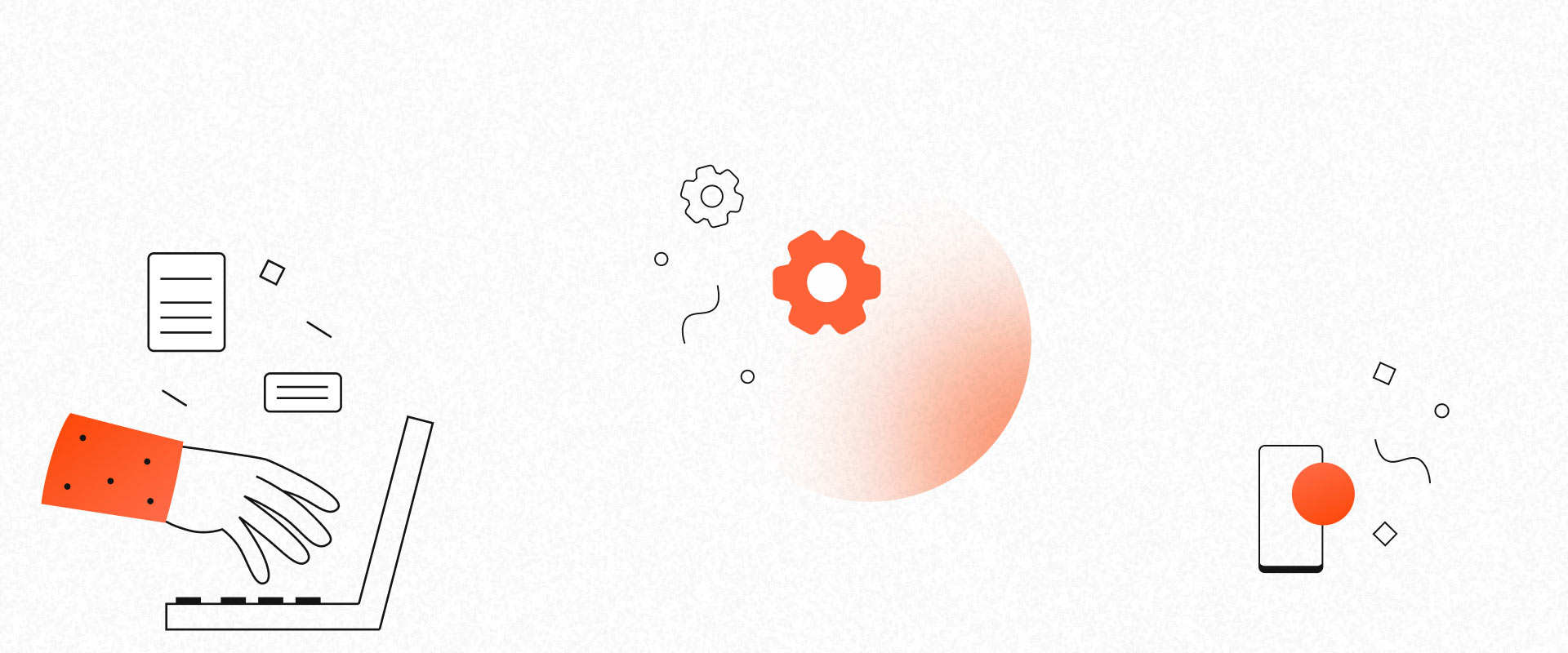
Have you ever imagined someone else doing your job for you? Surely you did, I know I have. So, today I bring some good news — your dreams can become reality! But, to make those dreams come true, you will need some knowledge of OOP (Object Oriented Programming) and a few tools, which I will talk about later. At COBE, we use Java, so before we start, I would suggest you get a book called Head First Java. Or if you are more of a visual type of person, like me, I would recommend a free course from Udemy. If you are already planning on giving up, please don’t. I will lead you through the whole setup of a project, and I am sure that at the end of this article you will be able to write your first automated test. So let’s go!
Why are automated tests performed at all? With each change or new implementation in a project, we as QA engineers have to go through the entire application to determine if the changes or new features have caused unexpected defects on parts that have worked perfectly so far. This way we:
1. Save time and money 💰
2. Achieve greater accuracy in conducting tests (the human error factor is inevitable) 🎯
3. Insure ourselves against repetitive actions 🔁
Imagine a project that takes several years, and you have to go through parts of the application that you have already seen 1000 times over and over again every few days? It doesn’t sound fun at all to me, and to you? I don’t think so.
In order to even begin the process of developing automated tests, you need to master the manual testing technique. I know, I wasn’t too happy about it either, but as soon as I realized that if I want to develop a quality automated test, I need to know everything about the application I’m working with. And the only way to achieve that is to test it manually first. Okay, now that you know why, let’s move on to how.
The first thing you need to install is Java. Download the latest version of the JDK (Java Development Kit), depending on the operating system you are using. Once you have successfully installed it, you need to set it as an environment variable. If you’re a macOS user, you can find the instructions at this link, and if you use Windows, find instructions here.
The next thing you need is an IDE (Integrated Development Environment). That’s where you will develop your code. In practice, people mostly use Eclipse and IntelliJ IDEA. At COBE, we use IntelliJ, so the examples that will follow, after we go through the whole setup, will be developed in it. The installation is fairly simple, but if you still want someone to walk you through it, you can find instructions here. The narrator of the instructions is nothing compared to Sir David Attenborough, but I believe you will be satisfied anyways when you develop your first automated test.
After a successful installation, it is necessary to create a new project. After clicking on Create new project, you need to select Maven located on the left side of the window, and finally you need to select the project name and press Finish.
Selenium is a framework for testing web applications. We will use it to retrieve the elements and finally choose what we want to do with those elements. Selenium .jar files can be easily added to our project using the pom.xml file generated at the time you created your project, and this is one of the main advantages of Maven.
In the pom.xml file we need to add a <dependencies> category to which we will add the following:
Once you have added the dependency group for Selenium (which you can also find here), you need to load the changes you made in Maven. You can do this by clicking on the icon pointed to by the arrow in the image above or by using a shortcut on the Mac, Shift + Command + I, while you are inside the pom.xml file.
TestNG is a framework used in Java, and we will use it to validate tests. It needs to be added, like Selenium, through the pom.xml file, and its dependency group looks like this:
Or you can find it here. While I have a feeling I’m repeating myself (repetition is the mother of knowledge), don’t forget to load the changes you’ve made in Maven. 👨🏫
The last thing we need before we start writing our code is WebDriver. It will allow us to open the desired web pages in the browser, to send various user inputs, execute JavaScript methods, etc. We will use ChromeDriver, which you can download here. For those of you who don’t prefer Chrome, there are WebDrivers for Firefox and Internet Explorer, while Safari has a native driver that you can turn on (because it’s turned off by default) through the terminal using the following command:
It is important to make sure that the versions of WebDriver you are downloading and your installed browser match!
Once you’ve downloaded WebDriver, move it to an easily accessible location, as we’ll need its path when we start writing your first automated test.
Before you start writing your code, you need to open the IntelliJ IDEA Preferences and in the section Build, Execution, Deployment open the Compiler folder, then click on Java Compiler, and when you’re in it, change the Target bytecode version to 8 as shown in the figure below:
First step you need to do is create a class. We will create the class in the way shown in the image below:
You need to open the test folder, and then a right click on the java subfolder. Under the New category, select Java Class. Once you have assigned the desired name to the class, press Enter.
Then you need to initialize WebDriver and set the URL of the page you want to open. In practice, it looks like this:
It is necessary to specify which WebDriver it is, and forward the path on which it is located. Using driver.get(), we retrieve the desired page. Now that you have enabled WebDriver and opened the website, you can start inspecting the elements you want to work with. Eg. If you have opened the cobe.tech page and you want to click on the Blog section in the navigation, you need to find something specific for it, so that Selenium knows exactly what you want to open. By right clicking and selecting the Inspect option on the element, a window will open, as you can see in the picture below:
The element you are looking for is framed in the image, but it is also by nature highlighted so you know exactly what you are inspecting. In case of too little information, you can use the ChroPath addon through which you can get the xPath to your element. In practice, it would look something like this:
Using this block of code, we found our element via xPath and ordered Selenium to click on the element. We also defined a time limit of 15 seconds in which the element must appear, otherwise the test fails because the required element was not found.
Why did we do that? 🤔 It’s just that sometimes pages take a little longer to load data, and even so we’ve avoided using the Thread.sleep() method, which forcibly pauses code execution. Otherwise you can search for elements through different things, but I will leave that to you to discover for yourself. In addition to the click() method, the second most commonly used method is sendKeys(), which, as the name suggests, allows you to send characters to a field.
Finally, to know if your test passed or failed, we will use the method from the TestNG framework. It works on the principle that you say what you expect, and then compare it to the actual value. As in our example, we want to check if we are really on the Blog section. At the beginning of this article, I mentioned that the path to successful automated tests is paved with manual testing, because in this case we wouldn’t know what to expect if manual testing hadn’t been conducted before. We can take the word Development as the expected value, as this is the name of one of the filters in the Blog section. For the actual value we take the location of the element Development and use get.text() method to retrieve it, which will look like this:
After running your first automated test, you will get a short printout in the console where you will be able to find out how many tests you have conducted, how many of them passed and how many failed. And that’s it. You did it! Great job!
So, how did you like everything you read so far? Did I encourage you to try automated testing yourself? And don’t worry, once you master the basics, you’ll be able to dig even deeper into the world of automated testing. Maybe we’ll meet there. 😄
If you’re still looking to find courage, I challenge you to write your first automated test using the guidelines I gave you. You can do a subscription test and check the functionality of our Newsletter section, where you will have the opportunity to use the sendKeys() method, but also explore new things yourself, as you will need a JavaScript method that will scroll to our Newsletter section. Don’t be discouraged because Katarina adores when someone subscribes to the Newsletter, and she will be even happier if she knows you did it through an automated test. #TrueStory
A little encouragement for the end — I never saw myself writing an article for the public to see it, but it happened. So if you thought you would never develop automated tests, guess what? Now is the time. All you need is a little faith. 🍀
And hey, don’t be a stranger, if you have any questions, feel free to contact me.
Ivan Hmelik is a Quality Assurance Engineer. In addition to dealing with manual testing, he has strong interest in the field of developing automated tests. Outside of the Quality Assurance world, he loves hanging out with friends and watching sports.